More messing around with ChatGPT and automating documentation for APIs. This time, instead of using a JSON file, I used a description of one of the entities in Azure Media Services.
I also installed the PlantUML plugin on this website. The rendered chart is below the code sample. It’s not exactly right, as an asset is really a storage container that extends the storage container with its own properties and methods. It’s a start though.
Test 1
PROMPT
1. Determine the entities in the following text: An Asset is mapped to a blob container in the Azure Storage account and the files in the Asset are stored as block blobs in that container. Assets contain information about digital files stored in Azure Storage (including video, audio, images, thumbnail collections, text tracks, and closed caption files).
2. Describe the relationship of the entities in PlantUML.
RESULTS
@startuml
class Asset {
+info: String
}
class BlobContainer {
}
class AzureStorage {
}
class File {
}
class BlockBlob {
}
class Video {
}
class Audio {
}
class Image {
}
class ThumbnailCollection {
}
class TextTrack {
}
class ClosedCaptionFile {
}
Asset –> BlobContainer
BlobContainer –> AzureStorage
Asset –> File
File –> BlockBlob
Asset –> Video
Asset –> Audio
Asset –> Image
Asset –> ThumbnailCollection
Asset –> TextTrack
Asset –> ClosedCaptionFile
@enduml
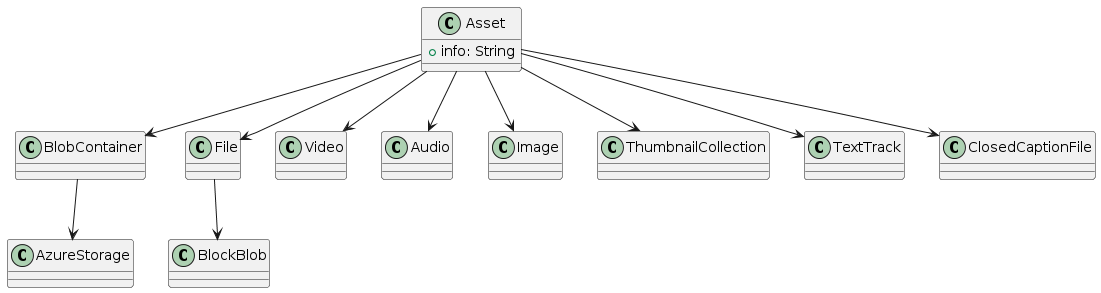
TEST 2
This time, I tried using a truncated version of the Asset JSON for Azure Media Services.
I got the following feedback from ChatGPT about the formatting of the JSON, which I appreciate.
“This diagram shows that “Asset” class has an attribute “AssetProperties” which is an object of type “AssetProperties”, which in turn has an attribute “properties” that is an object of “Properties”. And the “Properties” class has several attributes, including “assetId”, “created”, “lastModified”, “alternateId”, “description”, “container”, “storageAccountName”, and “storageEncryptionFormat”. It should be noted that the JSON provided has duplicate information and also the properties types are not well formatted to be used in PlantUML.”
PROMPT
Create a PlantUML class diagram using the following JSON:
{
"Asset" : {
"AssetProperties": {
"properties": {
"assetId": {
"type": "string",
"format": "uuid",
"description": "The Asset ID.",
"readOnly": true,
"x-nullable": false
},
"created": {
"type": "string",
"format": "date-time",
"description": "The creation date of the Asset.",
"readOnly": true,
"x-nullable": false
},
"lastModified": {
"type": "string",
"format": "date-time",
"description": "The last modified date of the Asset.",
"readOnly": true,
"x-nullable": false
},
"alternateId": {
"type": "string",
"description": "The alternate ID of the Asset."
},
"description": {
"type": "string",
"description": "The Asset description."
},
"container": {
"type": "string",
"description": "The name of the asset blob container."
},
"storageAccountName": {
"type": "string",
"description": "The name of the storage account."
},
"storageEncryptionFormat": {
"type": "string",
"enum": [
"None",
"MediaStorageClientEncryption"
],
"x-ms-enum": {
"name": "AssetStorageEncryptionFormat",
"values": [
{
"value": "None",
"description": "The Asset does not use client-side storage encryption (this is the only allowed value for new Assets)."
},
{
"value": "MediaStorageClientEncryption",
"description": "The Asset is encrypted with Media Services client-side encryption."
}
],
"modelAsString": true
},
"description": "The Asset encryption format. One of None or MediaStorageEncryption.",
"readOnly": true,
"x-nullable": false
}
},
"type": "object",
"description": "The Asset properties."
}
}
}
RESULTS
Here’s the resulting PlantUML:
@startuml
class Asset {
-AssetProperties : AssetProperties
}
class AssetProperties {
-properties : Properties
-type : object
-description : string
}
class Properties {
-assetId : string
-created : string
-lastModified : string
-alternateId : string
-description : string
-container : string
-storageAccountName : string
-storageEncryptionFormat : string
}
@enduml
Here’s the class diagram:
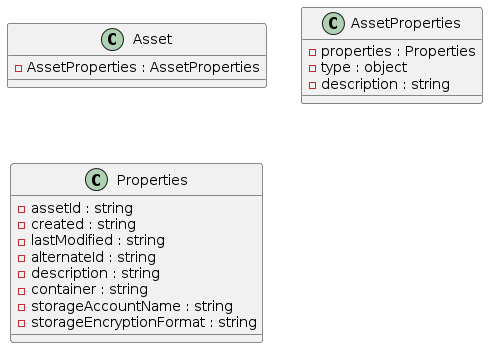